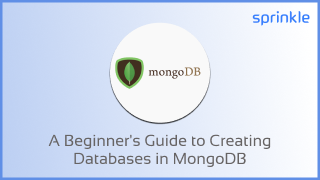
A Beginner's Guide to Creating Databases in MongoDB
MongoDB is a popular NoSQL database that allows for flexible and scalable data storage. It is known for its ease of use and flexibility, making it a preferred choice for many developers and organizations. In this article, we will walk you through the process of creating databases in MongoDB, step by step.
What is MongoDB?
MongoDB is a document-oriented NoSQL database that stores data in a flexible, JSON-like format called BSON (Binary JSON). Unlike traditional relational databases, MongoDB does not require a predefined schema, allowing you to store data in a more dynamic and adaptable manner. It is well-suited for a wide range of applications, from simple web applications to complex, data-intensive systems.
Installation and Setup
Before you can create databases in MongoDB, you need to have MongoDB installed on your system. You can download and install MongoDB from the official website (https://www.mongodb.com/try/download/community).
Note: The MongoDB Community Edition is now free and open-source, and it can be downloaded from the MongoDB website or from popular package managers such as apt and yum.
Once MongoDB is installed, you can start the MongoDB server by running the following command in your terminal:
"
mongosh
"
This will start the MongoDB server, and you'll be ready to create databases.
Creating a Database
To create a new database in MongoDB, you can use the use command followed by the name of the database you want to create. For example, to create a database named "mydatabase," you can run the following command:
"
use mydatabase
"
Note: The use command is not required to create a database in MongoDB. If you insert data into a non-existent database, MongoDB will create the database for you automatically.
Inserting Data
Now that you have created your database, you can start inserting data into it. MongoDB stores data in collections, which are similar to tables in relational databases. You can create a collection and insert data into it using the following commands:
db.createCollection("mycollection");
db.mycollection.insertOne({ name: "John", age: 30 });
db.mycollection.insertOne({ name: "Jane", age: 25 });
In the example above, we first create a collection named "mycollection" using the createCollection() method. Then, we insert two documents (data entries) into the collection using the insertOne() method.
Managing Your Database
MongoDB provides various commands for managing your databases. Some common tasks include listing the available databases, switching between databases, and dropping (deleting) databases.
To list the available databases, you can run:
"
show dbs
"
To switch to a different database, you can use the use command, as mentioned earlier.
To drop a database, be cautious as this action is irreversible and will permanently delete all data in the database. Use the following command:
db.adminCommand({dropDatabase: 1})
Note: The dropDatabase() command is now deprecated.
Here’s some additional information that will help you:
MongoDB Basics
Document-Oriented Data Model
MongoDB's document-oriented data model allows you to store data in a way that closely resembles JSON objects. Each document can have varying structures, which means you can store data without worrying about rigid schemas. This flexibility is particularly useful in situations where data is rapidly changing or when dealing with semi-structured or unstructured data.
Documents in MongoDB can be nested, allowing you to store complex data relationships in a single document. For example, you could store a user document with an embedded array of addresses. This would allow you to easily query for the user's addresses without having to perform multiple joins.
Collections
Collections in MongoDB are where documents are stored. Unlike tables in traditional relational databases, collections do not enforce a fixed schema. Documents within the same collection can have different fields, making it easy to adapt to evolving data requirements.
Collections are not limited to a single shard. When sharding is enabled, MongoDB will distribute documents across multiple shards based on the shard key. This allows you to scale your database to handle large datasets.
CRUD Operations
MongoDB supports a wide range of operations for working with data:
- Create (Insert): You can use the insertOne or insertMany method to add data to a collection. This allows for both single document and batch insertions.
- Read (Query): MongoDB offers powerful querying capabilities using methods like find. Queries can include conditions, projections, and sorting options to retrieve precisely the data you need.
- Update: To modify existing documents, you can use updateOne or updateMany. These methods allow you to selectively update fields within documents.
- Delete: MongoDB provides deleteOne and deleteMany methods to remove documents from a collection.
- MongoDB also supports atomic transactions, which allow you to perform multiple CRUD operations on multiple documents as a single unit.
MongoDB Databases and Data Modeling
Schema Validation
MongoDB 3.2 and later versions support schema validation, allowing you to define rules for data integrity. You can specify data types, required fields, and custom validation logic to ensure the data adheres to your application's requirements.
MongoDB 4.4 and later versions also support JSON schema validation. This allows you to define and validate your database schema using JSON Schema documents.
Indexing
Indexing is crucial for optimizing query performance. MongoDB allows you to create various types of indexes, such as single-field, compound, and geospatial indexes. Proper indexing can significantly accelerate query execution by enabling MongoDB to locate data efficiently.
Data Modeling Best Practices
Effective data modeling in MongoDB involves carefully designing document structures to match your application's query patterns. Consider embedding related data for better query performance and minimize the need for complex joins. However, it is generally recommended to avoid embedding large or complex documents in other documents, as this can lead to performance issues and make it difficult to maintain your data model.
Creating Databases and Collections
Access Control and Authentication
Securing your MongoDB databases is essential. You can set up user authentication to control who can access your data. MongoDB provides role-based access control (RBAC), allowing you to grant specific permissions to users or roles. You can also implement RBAC at the collection level to grant specific permissions to users or roles on individual collections.
Database Naming Conventions
Adhering to consistent naming conventions for databases and collections can enhance collaboration and organization within your development team. Choose meaningful, descriptive names that reflect the purpose of the data they store. Avoid using special characters in database and collection names.
Storage Engines
MongoDB offers different storage engines. The most commonly used engine is WiredTiger, known for its data compression and performance improvements. In-memory storage engines are ideal for scenarios where data needs to be accessed quickly but doesn't need to persist beyond a server restart. However, in-memory storage engines are not suitable for production use cases where data needs to be persisted beyond a server restart.
Working with Data
Inserting Data
In addition to insertOne and insertMany, MongoDB provides advanced features for data insertion. For instance, you can use the Bulk Write Operations API for efficiently inserting large volumes of data.
MongoDB also supports the upsert operation, which allows you to insert a new document if it does not exist or update the existing document if it does exist.
Querying Data
MongoDB's query language is rich and expressive. You can filter data using comparison operators, logical operators, and regular expressions. Aggregation pipelines allow for complex transformations and aggregations of data.
When querying data, it is important to use efficient queries. For example, you should avoid using wildcards in your query expressions, as this can force MongoDB to scan the entire collection.
Updating and Deleting Data
Beyond simple updates and deletes, MongoDB offers features like upserts (insert if not found, update if found) and array manipulation operators, which can be particularly useful when working with nested arrays in documents.
For example, you could use the $push operator to add a new element to an array within a document. Or, you could use the $pull operator to remove an element from an array within a document.
Database Management and Optimization
Backing Up and Restoring Databases
MongoDB provides utilities like mongodump and mongorestore for creating and restoring database backups. Consider implementing regular backup strategies to protect against data loss.
MongoDB also supports point-in-time recovery (PITR), which allows you to restore your database to a specific point in time.
Sharding
Sharding is a horizontal scaling technique that distributes data across multiple servers or shards. It's essential for handling large datasets and high write loads. MongoDB's sharding capabilities allow you to scale your system as your data grows.
MongoDB also supports sharded replica sets, which provide high availability and scalability for large datasets.
Replica Sets
Replica sets are clusters of MongoDB servers that provide high availability and data redundancy. In the event of server failures, a replica set can automatically promote a secondary node to become the new primary, ensuring uninterrupted service.
MongoDB also supports electing a hidden member as the primary node in a replica set. This can be useful for disaster recovery scenarios.
MongoDB Best Practices
Data Modeling Best Practices
Effective data modeling in MongoDB involves carefully designing document structures to match your application's query patterns. Consider embedding related data for better query performance and minimize the need for complex joins. However, it is generally recommended to avoid embedding large or complex documents in other documents, as this can lead to performance issues and make it difficult to maintain your data model.
Query Optimization
Analyze query performance using the MongoDB profiler and identify slow-running queries. Proper indexing, efficient queries, and limit/skip optimization can significantly enhance performance.
Monitoring and Profiling
MongoDB provides tools like the MongoDB Monitoring Service (MMS) and built-in diagnostic tools for real-time monitoring and profiling. Monitor metrics like disk space usage, memory consumption, and query execution times to proactively identify and resolve issues.
Here are some code examples for common MongoDB operations:
Python
# Create a database
db = client["my_database"]
# Create a collection
collection = db["my_collection"]
# Insert a document
document = {"name": "John Doe", "age": 30}
collection.insert_one(document)
# Find all documents
results = collection.find({})
# Update a document
collection.update_one({"_id": document["_id"]}, {"$set": {"age": 31}})
# Delete a document
collection.delete_one({"_id": document["_id"]})
Common Mistakes to Avoid
- Not using indexes: Not using indexes can lead to slow query performance and increased resource usage.
- Overusing embedded documents: Overusing embedded documents can lead to performance issues and make it difficult to maintain your data model.
- Ignoring data validation: Ignoring data validation can lead to data corruption and errors in your application.
By following these best practices, you can avoid common mistakes and get the most out of MongoDB.
Here are some additional tips and resources for using MongoDB:
- Use the right storage engine for your needs: MongoDB offers different storage engines, each with its own strengths and weaknesses. Choose the storage engine that best meets the requirements of your application.
- Tune your database: MongoDB provides a variety of parameters that can be tuned to improve performance. Experiment with different parameter settings to find what works best for your application.
- Monitor your database: Use MongoDB's monitoring tools to track key metrics such as performance, disk usage, and memory consumption. This will help you to identify and resolve potential problems early on.
- Use the right tools: There are a number of third-party tools available to help you with MongoDB development and administration. For example, you can use a MongoDB IDE or GUI tool to make it easier to manage your database and perform common tasks.
Here are some resources that you may find helpful:
- MongoDB Documentation: https://www.mongodb.com/docs/
- MongoDB University: https://university.mongodb.com/
- MongoDB Blog: https://www.mongodb.com/blog/
- MongoDB Community Forums: https://forums.mongodb.com/
Conclusion
Creating databases in MongoDB is a straightforward process. With its flexibility and ease of use, MongoDB is an excellent choice for many types of applications. Remember that databases in MongoDB are created lazily, and you can start adding data to them once they are created. As you become more familiar with MongoDB, you can explore its advanced features and capabilities for handling complex data storage and retrieval tasks.