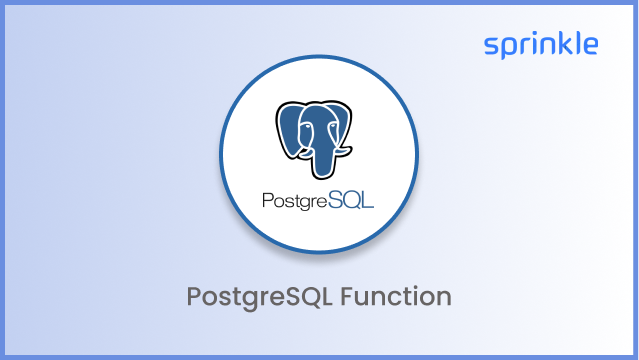
PostgreSQL is an enterprise-grade database system developed as an extension of the SQL language. Its code blocks, known as PostgreSQL functions, are stored procedures that allow users to define and perform specific tasks within the system. In a crux, it is known to be a user-defined procedural language in which users can create a user-defined function, redefine the existing function body, etc.
Functions stored in the database can be involved using the SQL interface but can be created using any language, including SQL, Python, Java, etc. However, the basic syntax of creating the specific function must be followed.
PostgreSQL Syntax
Consider the COUNT function in PostgreSQL. The function counts specific values in a table, non-null entries, or the number of rows and columns. The basic syntax for the COUNT function is:
SELECT COUNT(*) FROM table_name WHERE condition;
Where,
- SELECT is used to select data from a table.
- COUNT is the function used.
- The parentheses are used to specify the value for counting. The asterisk (*) in the above example denotes all the rows in the table. But it can also be substituted with a column or row name.
- table_name signifies the table from which values should be counted.
- WHERE gives a specific location or condition. Only those values which fulfill this condition are counted by the function.
Though more than 3000 functions are available in PostgreSQL, such as the PostgreSQL date functions. But one of the most commonly used functions is CREATE.
What is the PostgreSQL CREATE Function command?
The CREATE function is an inbuilt function that allows you to create a new, custom-defined function in PostgreSQL. Like all other functions, the CREATE function also has a syntax.
Syntax of PostgreSQL CREATE Function command
CREATE [OR REPLACE] FUNCTION function_name(param_list) RETURNS return_type LANGUAGE plpgsql AS $$ DECLARE -- variable declaration BEGIN -- logic END; $$
Each parameter has a specific function as explained in the table below.
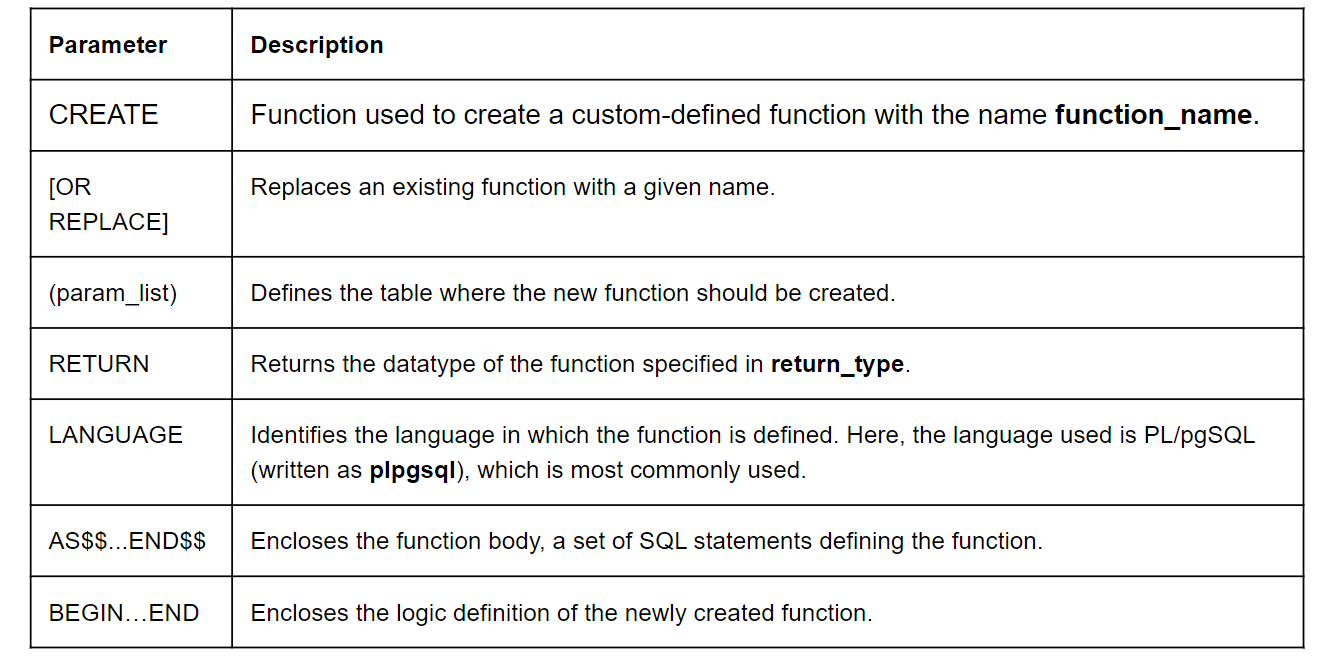
The CREATE function is a basic function commonly used in all PostgreSQL syntaxes. But to use PostgreSQL for database management, you must understand how these functions generally work.
How do PostgreSQL Functions work?
Each PostgreSQL function has an exact purpose different from all other functions in the database. However, all of them follow a basic workflow defined by three steps.
1. Creating a function
The CREATE function mentioned above is the first working step of a PostgreSQL function. You can use the CREATE function statement to define a new function that can be included in the PostgreSQL database.
Alternatively, you can use the REPLACE function statement to replace or redefine an existing function. Users have the flexibility to define their own functions also.
The syntax includes a unique name for the new function that isn't already in the database. The code also includes the input positional parameters and the return type before describing the logic of the new function.
A set of SQL statements is included in the function body, which acts as the logical definition of the function. These statements define the function's operation when it is called in a PostgreSQL code.
The syntax also has a language parameter to define the language in which the function is written. PostgreSQL supports many languages, such as SQL, C, Python, etc. However, if no language is specified, PostgreSQL assumes the code to be in the default language SQL.
2. Calling the function
You can use the newly created function in other codes by calling the function. When a function is called, it executes the SQL statements written in its logic part.
First, it captures the input values or parameters in the given code. These parameters define what the function operates on. For example, the function can perform addition or multiplication of the parameters.
But, the PostgreSQL code for enterprises is more complex than simple mathematical functions. That's why these codes have loops and structures. This allows the developers to use the same function to perform similar tasks in multiple ways.
LOOP, for instance, is a control function that allows you to repeat the same task repeatedly until a specific condition is met. This saves the hassle of retyping the same code for different parameters.
Once the code is executed, it moves to the next part: the return value.
3. Return
The "result” given back by the code to the database is the return value. The form of the return value is specified in the syntax by:
RETURN return_type
The return type returns integer, scalars, other simple values, or complex data structures.
Using the PRINT statement, you can display the return value as the result of the code. Or, you can use it as part of another code by assigning the return value to a variable.
The above three steps of the PostgreSQL function workflow can be better understood through some examples.
Examples of PostgreSQL Functions
In the below PostgreSQL function example, we create a new function that counts the number of students who scored between two defined scores. In the next example, we also delete a function irrelevant to the data table.
Example 1: CREATE FUNCTION
Code:
CREATE FUNCTION get_student_count (score_from int, score_to int) RETURNS int LANGUAGE plpgsql as $$ declare student_count integer; BEGIN SELECT count(*) into student_count from students where student_score between score_from and score_to; RETURN student_count; END; $$;
In the above code, we created a function “get_student_count” that returns the value as the number of students that scored between score_from and score_to. The language used is PL/pgSQL.
Example 2: Dropping a function
Let us create another function called student_number to get their roll numbers from the list.
CREATE function student_number() RETURNS int LANGUAGE plpgsql as $$ DECLARE count int; BEGIN SELECT count(*) into count from students; RETURN count; END; $$
However, our reference table has only student names and their scores. So the function student_number is irrelevant here and can be dropped.
To drop the function, we use the code:
DROP FUNCTION student_number;
This deletes the function student_number from the existing database. You can view all the available functions using the \df command.
The above examples are basic applications of the PostgreSQL functions. To create custom functions for your business, you must understand the different methods of creating a function in PostgreSQL.
Creating a function in PostgreSQL
There are two methods to create a function in PostgreSQL.
- Using pgAdmin
- Using SQL Shell
To help you choose the best method, we have provided a step-by-step guide for both methods below.
- Create a PostgreSQL function using pgAdmin
pgAdmin is an open-source administration and development platform specifically for PostgreSQL. Developers can create, manage, and interact with PostgreSQL databases through pgAdmin.
To create a function in pgAdmin:
Step 1: Launch the latest version of the pgAdmin tool and connect it to your PostgreSQL database. Here, we will name our database: Sprinkle.
Step 2: Navigate to the toolbar and click the Query Tool in the Tools dropdown menu.

Step 3: We will create a function get_car_Price. To create the function, type the code in the below screenshot in the Query Editor box. To run the code, click Execute.
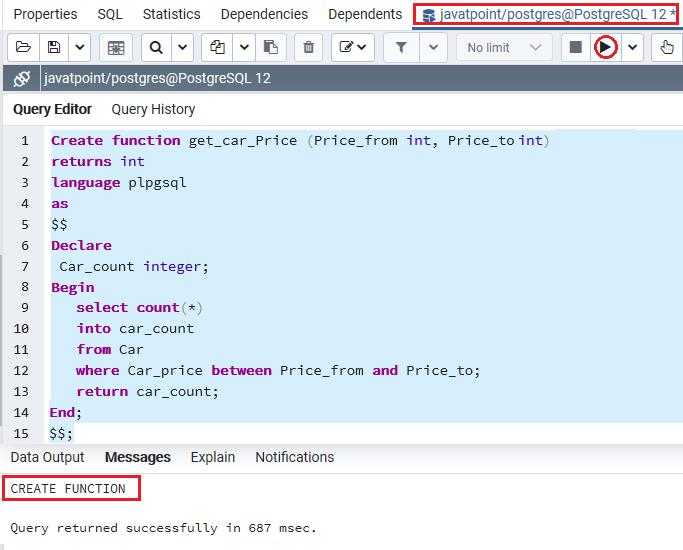
The message window at the bottom of the screen displays the status of the code. After execution, the message window displays that the function get_car_Price has been successfully created.
Step 4: You can check the function created by the above query in the Functions list under the browser tab at the side of the window.

If you cannot see the newly created function, right-click on the Functions menu and click on Refresh to update the list.
- Create a PostgreSQL function using SQL Shell
SQL Shell is a Dynamic SQL interface that allows you to develop and execute SQL commands.
To create a PostgreSQL function using SQL Shell, follow the below steps.
Step 1: Create a data table in the sprinkle database we created earlier.
Step 2: Launch the SQL Shell interface and connect the database using the following command.
- \c sprinkle
Step 3: Create a new function get_student_score1 using the below code.
sprinkle=# Create function get_student_score1(Price_from int, Price_to int) sprinkle-# returns int sprinkle-# language plpgsql sprinkle-# as sprinkle-# $$ sprinkle$# Declare sprinkle$# student_count integer; sprinkle$# Begin sprinkle$# select count(*) sprinkle$# into student_count sprinkle$# from student sprinkle$# where student_score between score_from and score_to; sprinkle$# return student_count; sprinkle$# End; sprinkle$# $$;
After executing the command, the function get_student_score1 will be successfully created.
Step 4: To check the newly created function, use the below command to get a list of all custom functions.
- sprinkle=# \df
Note: As we have already created the function get_student_score in the pgAdmin example using the same database, recreating the same function in SQL Shell gives an error. Instead, we have modified the new function name to get_student_score1.
PostgreSQL is a reliable database known for its architecture and data integrity. In this guide, we have explained PostgreSQL and its various functions in detail. We have also included step-by-step tutorials to create user-defined functions using two different tools. You can modify the above codes or implement your own to create and utilize PostgreSQL functions for your business.
Frequently Asked Questions FAQs - What is PostgreSQL function?
What are PostgreSQL functions?
PostgreSQL functions are stored procedures that allow users to perform specific tasks or calculations within the database. These functions can be written in various programming languages such as SQL, PL/pgSQL, Python, etc., and can accept input parameters and return values.
What are the basic functions of PostgreSQL?
The basic functions of PostgreSQL include mathematical operations, string manipulation functions, date/time functions, aggregate functions, and window functions.
What is any function in PostgreSQL?
In PostgreSQL, any function is a set of SQL statements that performs a specific task when called by other SQL statements or applications. Functions can be created using the CREATE FUNCTION statement and executed using the SELECT statement.
How many types of functions are there in PostgreSQL?
There are two types of functions in PostgreSQL: user-defined functions and built-in functions. Users create user-defined functions to perform custom tasks while PostgreSQL provides built-in functions for common tasks like mathematical calculations or string manipulations.
How do you list all functions in Postgres?
To list all functions in Postgres, you can use the following query:
SELECT proname FROM pg_proc WHERE pronamespace = 'public'::regnamespace;
What is the greatest feature of PostgreSQL?
One of the greatest features of PostgreSQL is its ability to create custom user-defined functions that can be used to extend the functionality of the database. This allows users to write complex logic in their preferred programming language and execute it within the database environment.
How many types of functions are there?
In SQL, there are several types of functions including scalar functions, table-valued functions, and aggregate functions. Each type serves a different purpose depending on the requirements of the query being executed.
What types does the Postgres function return?
Postgres function can return various data types such as integers, strings, arrays, records, and tables based on its definition.